Expand description
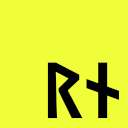
A stack-based virtual machine for the Rust programming language.
This is the driver for the Rune Language.
Re-exports§
pub use self::format::Format;
pub use self::format::FormatSpec;
pub use self::module::InstFnNameHash;
pub use self::module::InstallWith;
pub use self::module::Module;
pub use crate::debug::DebugInfo;
pub use crate::debug::DebugInst;
Modules§
- budget
- Budgeting module for Runestick.
- debug
- Debug information for units.
- format
- Types for dealing with formatting specifications.
- module
- Crate used for definint native modules.
- modules
- Public packages that can be used to provide functionality to virtual machines.
Macros§
- span
- Construct a span that can be used during pattern matching.
Structs§
- AnyObj
- Our own private dynamic Any implementation.
- AnyObj
Vtable - The vtable for any type stored in the virtual machine.
- Borrow
Mut - Guard for data exclusively borrowed from a slot in the virtual machine.
- Borrow
Ref - Guard for a data borrowed from a slot in the virtual machine.
- Byte
Index - A single index in a Span, like the start or ending index.
- Bytes
- A vector of bytes.
- Call
Frame - A call frame.
- Compile
Item - Item and the module that the item belongs to.
- Compile
Meta - Compile-time metadata about a unit.
- Compile
Meta Capture - Metadata about a closure.
- Compile
Meta Empty - The metadata about a type.
- Compile
Meta Struct - The metadata about a type.
- Compile
Meta Tuple - The metadata about a variant.
- Compile
Mod - Module, its item and its visibility.
- Compile
Source - Information on a compile sourc.
- Context
- Static run context visible to the virtual machine.
- Context
Type Info - Information on a specific type.
- Debug
Label - A label that can be jumped to.
- Future
- A type-erased future that can only be unsafely polled in combination with the virtual machine that created it.
- Generator
- A generator with a stored virtual machine.
- Hash
- The hash of a primitive thing.
- Id
- An opaque identifier that is associated with AST items.
- Item
- The name of an item.
- Iterator
- An owning iterator.
- Label
- A label that can be jumped to.
- Location
- A source location.
- Mut
- A strong mutable reference to the given type.
- Names
- A tree of names.
- NotAccessible
Mut - Error raised when tried to access for exclusive access but it was not accessible.
- NotAccessible
Ref - Error raised when tried to access for shared access but it was not accessible.
- Object
- Struct representing a dynamic anonymous object.
- Panic
- A descriptibe panic.
- Protocol
- A built in instance function.
- Range
- Struct representing a dynamic anonymous object.
- RawAccess
Guard - A raw guard around some level of access.
- RawMut
- A raw guard to a Ref.
- RawRef
- A raw guard to a Ref.
- RawStr
- A raw static string.
- Ref
- A strong reference to the given type.
- Rtti
- Runtime information on variant.
- Runtime
Context - Static run context visible to the virtual machine.
- Select
- A stored select.
- Shared
- A shared value.
- Shared
Pointer Guard - A guard for an
Any
containing a pointer. - Source
- A single source file.
- Span
- A span corresponding to a range in the source file being parsed.
- Spanned
Error - An error with an associated span.
- Stack
- The stack of the virtual machine, where all values are stored.
- Stack
Error - An error raised when interacting with the stack.
- Static
String - Struct representing a static string.
- Static
Type - Static type information.
- Stream
- A stream with a stored virtual machine.
- Struct
- An object with a well-defined type.
- Tuple
- Struct representing a dynamic anonymous object.
- Tuple
Struct - A tuple with a well-defined type.
- Unit
- Instructions from a single source file.
- Unit
Struct - A empty with a well-defined type.
- Variant
- The variant of a type.
- Variant
Rtti - Runtime information on variant.
- Vec
- Struct representing a dynamic vector.
- VecTuple
- A helper type to deserialize arrays with different interior types.
- Vm
- A stack which references variables indirectly from a slab.
- VmCall
- An instruction to push a virtual machine to the execution.
- VmError
- Errors raised by the execution of the virtual machine.
- VmExecution
- The execution environment for a virtual machine.
- VmInteger
Repr - A type-erased rust number.
- VmSend
Execution - A wrapper that makes
VmExecution
Send
.
Enums§
- Access
Error - An error raised while downcasting.
- AnyObj
Error - Errors raised during casting operations.
- Awaited
- A stored await task.
- Call
- How the function is called.
- Compile
Meta Kind - Compile-time metadata kind about a unit.
- Component
- The component of an item.
- Component
Ref - A reference to a component of an item.
- Const
Value - A constant value.
- Context
Error - An error raised when building the context.
- Context
Signature - A description of a function signature.
- Generator
State - The state of a generator.
- Inst
- An operation in the stack-based virtual machine.
- Inst
Address - How an instruction addresses a value.
- Inst
Assign Op - An operation between two values on the machine.
- InstOp
- An operation between two values on the machine.
- Inst
Range Limits - Range limits of a range expression.
- Inst
Target - The target of an operation.
- Inst
Value - A literal value that can be pushed.
- Inst
Variant - A variant that can be constructed.
- Key
- A key that can be used as an anonymous object key.
- Panic
Reason - Pre-canned panic reasons.
- Range
Limits - The limits of a range.
- Type
Check - An encoded type check.
- Type
Info - Type information about a value, that can be printed for human consumption through its Display implementation.
- UnitFn
- The kind and necessary information on registered functions.
- Value
- An entry on the stack.
- Variant
Data - The data of the variant.
- Visibility
- Information on the visibility of an item.
- VmError
Kind - The kind of error encountered.
- VmHalt
- The reason why the virtual machine execution stopped.
- VmHalt
Info - The reason why the virtual machine execution stopped.
Statics§
- BOOL_
TYPE - The specialized type information for a bool type.
- BYTES_
TYPE - The specialized type information for a bytes type.
- BYTE_
TYPE - The specialized type information for a byte type.
- CHAR_
TYPE - The specialized type information for a char type.
- FLOAT_
TYPE - The specialized type information for a float type.
- FORMAT_
TYPE - The specialized type information for a fmt spec types.
- FUNCTION_
TYPE - The specialized type information for a function pointer type.
- FUTURE_
TYPE - The specialized type information for a future type.
- GENERATOR_
STATE_ TYPE - The specialized type information for a generator state type.
- GENERATOR_
TYPE - The specialized type information for a generator type.
- INTEGER_
TYPE - The specialized type information for a integer type.
- ITERATOR_
TYPE - The specialized type information for the iterator type.
- OBJECT_
TYPE - The specialized type information for an anonymous object type.
- OPTION_
TYPE - The specialized type information for a option type.
- RANGE_
TYPE - The specialized type information for the range type.
- RESULT_
TYPE - The specialized type information for a result type.
- STREAM_
TYPE - The specialized type information for the
Stream
type. - STRING_
TYPE - The specialized type information for a string type.
- TUPLE_
TYPE - The specialized type information for an anonymous tuple type.
- TYPE
- The specialized type information for type objects.
- UNIT_
TYPE - The specialized type information for a unit.
- VEC_
TYPE - The specialized type information for a vector type.
Traits§
- Any
- A trait which can be stored inside of an AnyObj.
- Args
- Trait for converting arguments onto the stack.
- From
Value - Trait for converting from a value.
- Guarded
Args - Trait for converting arguments onto the stack.
- Into
Byte Index - Convert the given type into an index.
- Into
Component - Trait for encoding the current type into a component.
- Into
Type Hash - Helper conversion into a function hash.
- Named
- Something that is named.
- ToValue
- Trait for converting types into values.
- TypeOf
- Trait used for Rust types for which we can determine the runtime type of.
- Unsafe
From Value - A potentially unsafe conversion for value conversion.
- Unsafe
ToValue - Trait for converting types into values.
- With
Span - Trait to coerce a result of a non-spanned error into a spanned error.
Type Aliases§
- Error
- Exported boxed error type for convenience.
- Function
- A callable non-sync function.
- Result
- Exported result type for convenience.
- Source
Id - The identifier of a source file.
- Sync
Function - A callable sync function. This currently only supports a subset of values that are supported by the Vm.