Expand description
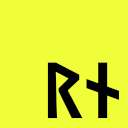
Minimum support: Rust 1.81+.
Visit the site π — Read the book π
The Rune Language, an embeddable dynamic programming language for Rust.
Β§Contributing
If you want to help out, please have a look at Open Issues.
Β§Highlights of Rune
- Runs a compact representation of the language on top of an efficient stack-based virtual machine.
- Clean Rust integration π».
- Multithreaded π execution.
- Hot reloading π.
- Memory safe through reference counting π.
- Awesome macros π and Template literals π.
- Try operators π and Pattern matching π.
- Structs and enums π with associated data and functions.
- Dynamic containers like vectors π, objects π, and tuples π all with out-of-the-box serde support π».
- First-class async support π with Generators π.
- Dynamic instance functions π.
- Stack isolation π between function calls.
Β§Rune scripts
You can run Rune programs with the bundled CLI:
cargo run --bin rune -- run scripts/hello_world.rn
If you want to see detailed diagnostics of your program while itβs running, you can use:
cargo run --bin rune -- run scripts/hello_world.rn --dump --trace
See --help
for more information.
Β§Running scripts from Rust
You can find more examples in the
examples
folder.
The following is a complete example, including rich diagnostics using
termcolor
. It can be made much simpler if this is not needed.
use rune::{Context, Diagnostics, Source, Sources, Vm};
use rune::termcolor::{ColorChoice, StandardStream};
use std::sync::Arc;
let context = Context::with_default_modules()?;
let runtime = Arc::new(context.runtime()?);
let mut sources = Sources::new();
sources.insert(Source::memory("pub fn add(a, b) { a + b }")?);
let mut diagnostics = Diagnostics::new();
let result = rune::prepare(&mut sources)
.with_context(&context)
.with_diagnostics(&mut diagnostics)
.build();
if !diagnostics.is_empty() {
let mut writer = StandardStream::stderr(ColorChoice::Always);
diagnostics.emit(&mut writer, &sources)?;
}
let unit = result?;
let mut vm = Vm::new(runtime, Arc::new(unit));
let output = vm.call(["add"], (10i64, 20i64))?;
let output: i64 = rune::from_value(output)?;
println!("{}", output);
ModulesΒ§
- alloc
- The Rune core allocation and collections library
- ast
- Abstract syntax trees for the Rune language.
- cli
cli
- Helper to build customized commandline interfaces using custom rune contexts.
- compile
- The Rune compiler.
- diagnostics
- Diagnostics module for Rune.
- fmt
- Helper to format Rune code.
- hash
- Utilities for working with hashes.
- item
- Types related to items.
- languageserver
- Utility for building a language server.
- macros
- The macro system of Rune.
- module
- Types used for defining native modules.
- modules
- Public packages that can be used to provide extract functionality to virtual machines.
- no_std
- Helper prelude for
#[no_std]
support. Public types related to using rune in #no_std environments. - parse
- Parsing utilities for Rune.
- query
- Lazy query system, used to compile and build items on demand and keep track of whatβs being used and not.
- runtime
- Runtime module for Rune.
- source
- Module for dealing with sources.
- termcolor
emit
- This crate provides a cross platform abstraction for writing colored text to a terminal. Colors are written using either ANSI escape sequences or by communicating with a Windows console. Much of this API was motivated by use inside command line applications, where colors or styles can be configured by the end user and/or the environment.
- workspace
workspace
- Types for dealing with workspaces of rune code.
MacrosΒ§
- K
- Helper macro to reference a specific token kind, or short sequence of kinds.
- T
- Helper macro to reference a specific token.
- docstring
- Convenience macro for extracting a documentation string from documentation comments.
- hash
- Calculate a type hash at compile time.
- item
- Calculate an item reference at compile time.
- sources
- Helper macro to define a collection of sources populatedc with the given entries.
- static_
env - Defines a static budget and environment implementation suitable for
singlethreaded no-std environments. This can be used in
#[no_std]
environments to implement the necessary hooks for Rune to work. - vm_
panic - Helper to cause a panic.
- vm_try
- Helper to perform the try operation over
VmResult
. - vm_
write - Helper macro to perform a
write!
in a context which errors withVmResult
and returnsVmResult<Result<_, E>>
on write errors.
StructsΒ§
- Build
- A builder for a Unit.
- Build
Error - Error raised when we failed to load sources.
- Context
- Context used for the Rune language.
- Diagnostics
- Structure to collect compilation diagnostics.
- Hash
- The primitive hash that among other things is used to reference items, types, and native functions.
- Item
- The reference to an ItemBuf.
- ItemBuf
- The name of an item in the Rune Language.
- Module
- A Module that is a collection of native functions and types.
- Mut
- A strong owned mutable reference to the given type that can be safely dereferenced.
- Options
- Options that can be provided to the compiler.
- Params
- Helper to register a parameterized function.
- Ref
- A strong owned reference to the given type that can be safely dereferenced.
- Source
- A single source file.
- Source
Id - The opaque identifier of a source file, as returned by
Sources::insert
. - Sources
- A collection of source files.
- Unit
- Instructions and debug info from a single compilation.
- Value
- An entry on the stack.
- Vm
- A stack which references variables indirectly from a slab.
EnumsΒ§
- Context
Error - An error raised when building the context.
TraitsΒ§
- Any
- Derive for types which can be used inside of Rune.
- From
Value - Trait for converting types from the dynamic Value container.
- ToConst
Value - Convert a value into a constant value.
- ToType
Hash - Helper trait used to convert a type into a type hash.
- ToValue
- Trait for converting types into the dynamic
Value
container. - Type
Hash - Static type hash for a given type.
FunctionsΒ§
- from_
const_ value - Convert something into a
ConstValue
. - from_
value - Convert something into the dynamic
Value
. - prepare
- Entry point to building a collection
Sources
of Rune into a default executableUnit
. - to_
const_ value - Convert something into a
ConstValue
. - to_
value - Convert something into the dynamic
Value
.
Attribute MacrosΒ§
- function
- Macro used to annotate native functions which can be loaded into rune.
- module
- Macro used to annotate a module with metadata.
Derive MacrosΒ§
- Any
- Macro to mark a value as external, which will implement all the appropriate traits.
- From
Value - Derive macro for the
FromValue
trait for converting types from the dynamicValue
container. - ToConst
Value - Derive for the
ToConstValue
trait. - ToValue
- Derive macro for the
ToValue
trait for converting types into the dynamicValue
container.