Expand description
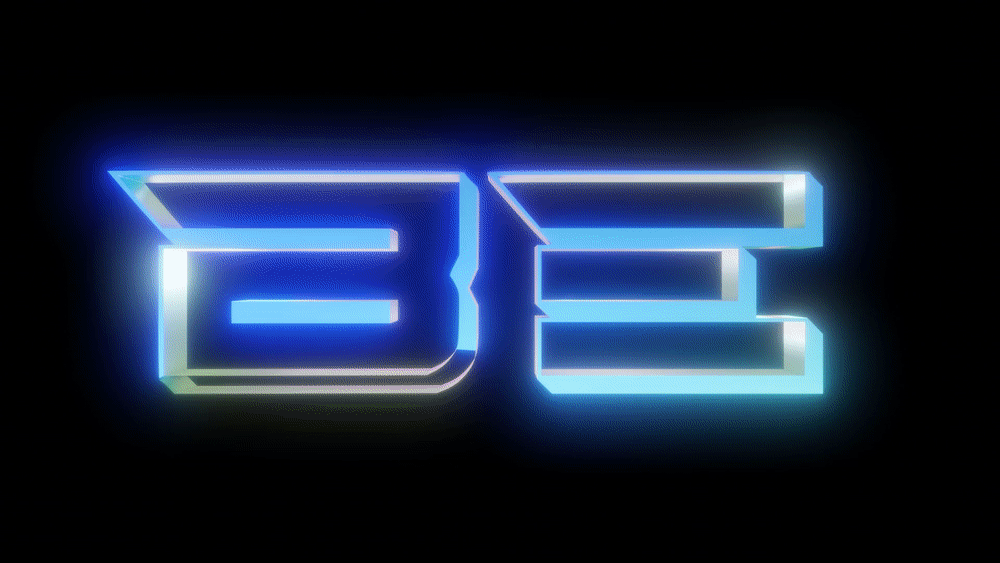
§Blue Engine
Blue Engine is an easy to use, portable, and extendable/customizable graphics engine. Here lives the documentation for the engine.
§Setup
The setup and installation details live in the project’s guide. A basic program in Blue Engine is as follow:
§Example
use blue_engine::{
prelude::{ Engine, ObjectSettings },
primitive_shapes::triangle
};
fn main() {
// initialize the engine
let mut engine = Engine::new();
// create a triangle
triangle("my triangle", ObjectSettings::default(), &mut engine.renderer, &mut engine.objects);
// run the engine
engine
.update_loop(move |_, _, _, _, _, _| {});
}
§Utilities
This crate is the core of the engine, but there is also utilities crate which have a lot of utilities for the engine such as lighting, physics, etc.
§Guide for code navigation
The code of the engine is organized in a rather different manner than traditional in the language. There are inspirations from other languages to make it easier to navigate the project.
§Older hardware
The engine uses WGPU under the hood for rendering. WGPU by nature is designed for modern hardware, so if you have or want to target older hardware, you might need to add a couple more things to EngineSettings during Engine::new_config:
- set a backend that targets your older hardware, such as GL using the backends field:
backend: blue_engine::wgpu::Backends::GL
- experiement with the limits field, which describes what features you need.
limits: blue_engine::wgpu::Limits::default()
. there are three options for limits:default
for normal hardware,downlevel_defaults
which are compatible with GLES-3 and D3D-11, ordownlevel_webgl2_defaults
which is also compatible with WebGL2, and the lowest level for limits and can support very old hardware.
with these two changes, hopefully you can get Blue Engine to run on older hardware. If not, please let me know so I can help you further.
Modules§
- engine
- contains defintions of top level functionality of the Engine
- error
- interal error definitions of the engine
- objects
- contains the definition for Object type, which is a type that make it easier to manage data for rendering.
- prelude
- contains all the declarations such as structs, exports, enums, …
- render
- contains definition for rendering part of the engine.
- utils
- Utilities for the engine (soon moving to it’s own crate).
- window
- contains definition for creation of window and instance creation.
Structs§
- Backends
- Represents the backends that wgpu will use.
- Camera
- Container for the camera feature. The settings here are needed for algebra equations needed for camera vision and movement. Please leave it to the renderer to handle
- Camera
Container - Container for Cameras
- Command
Encoder - Encodes a series of GPU operations.
- Engine
- The engine is the main starting point of using the Blue Engine. Everything that runs on Blue Engine will be under this struct. The structure of engine is monolithic, but the underlying data and the way it works is not. It gives a set of default data to work with, but also allow you to go beyond that and work as low level as you wish to.
- Engine
Settings - Descriptor and settings for a window.
- Event
Loop - Provides a way to retrieve events from the system and from the windows that were registered to the events loop.
- Input
Helper - main struct
- Instance
- Instance buffer data storage
- Instance
Raw - Instance buffer data that is sent to GPU
- KeyEvent
- Describes a keyboard input targeting a window.
- Logical
Insets - The logical distance between the edges of two rectangles.
- Logical
Position - A position represented in logical pixels.
- Logical
Size - A size represented in logical pixels.
- Logical
Unit - A logical pixel unit.
- Object
- Objects make it easier to work with Blue Engine, it automates most of work needed for creating 3D objects and showing them on screen. A range of default objects are available as well as ability to customize each of them and even create your own! You can also customize almost everything there is about them!
- Object
Settings - Extra settings to customize objects on time of creation
- Object
Storage - A unified way to handle objects
- Operations
- Pair of load and store operations for an attachment aspect.
- Physical
Insets - The physical distance between the edges of two rectangles.
- Physical
Position - A position represented in physical pixels.
- Physical
Size - A size represented in physical pixels.
- Physical
Unit - A physical pixel unit.
- Pipeline
- Container for pipeline values. Each pipeline takes only 1 vertex shader, 1 fragment shader, 1 texture data, and optionally a vector of uniform data.
- Render
Pass Color Attachment - Describes a color attachment to a
RenderPass
. - Render
Pass Descriptor - Describes the attachments of a render pass.
- Renderer
- Main renderer class. this will contain all methods and data related to the renderer
- Shader
Settings - These definitions are taken from wgpu API docs
- Signal
Storage - Handles the live events in the engine
- Texture
View - Handle to a texture view.
- Vertex
- Will contain all details about a vertex and will be sent to GPU
- Vertex
Buffers - Container for vertex and index buffer
- Window
- A wrapper for winit window to make it easier to use and more ergonomic.
Enums§
- Device
Event - Represents raw hardware events that are not associated with any particular window.
- Element
State - Describes the input state of a key.
- Event
- Describes a generic event.
- Fullscreen
- Fullscreen modes.
- Insets
- Insets that are either physical or logical.
- Key
- Key represents the meaning of a keypress.
- KeyCode
- Code representing the location of a physical key
- LoadOp
- Operation to perform to the output attachment at the start of a render pass.
- Memory
Hints - Hints to the device about the memory allocation strategy.
- Mouse
Button - Describes a button of a mouse controller.
- Pipeline
Data - Container for pipeline data. Allows for sharing resources with other objects
- Pixel
Unit - A pixel unit that’s either physical or logical.
- Position
- A position that’s either physical or logical.
- Projection
- Container for the projection used by the camera
- Rotate
Amount - Defines how the rotation amount is
- Rotate
Axis - Defines how the rotation axis is
- Size
- A size that’s either physical or logical.
- Texture
Data - Defines how the texture data is
- Texture
Mode - Defines how the borders of texture would look like
- Window
Event - Describes an event from a [
Window
].
Constants§
- DEPTH_
FORMAT - Depth Format
Traits§
- Pixel
- Pod
- Marker trait for “plain old data”.
- Signal
- Allows all events to be fetched directly, making it easier to add custom additions to the engine.
- Zeroable
- Trait for types that can be safely created with
zeroed
.
Functions§
- pixel_
to_ cartesian - This function helps in converting pixel value to the value that is between -1 and +1
- validate_
scale_ factor - Checks that the scale factor is a normal positive
f64
.
Type Aliases§
- Cull
Mode - Face of a vertex.
- Front
Face - Vertex winding order which classifies the “front” face of a triangle.
- Index
Format - Format of indices used with pipeline.
- Matrix2
- A 2x2 column major matrix.
- Matrix3
- A 3x3 column major matrix.
- Matrix4
- A 4x4 column major matrix.
- Polygon
Mode - Type of drawing mode for polygons
- Power
Preference - Power Preference when choosing a physical adapter.
- Quaternion
- A quaternion representing an orientation.
- Shader
Primitive - Primitive type the input mesh is composed of.
- Shaders
- Shaders are programs that runs on the GPU
- Textures
- Textures are image data that are sent to GPU to be set to a surface
- Uniform
Buffers - Uniform Buffers are small amount of data that are sent from CPU to GPU
- Unsigned
IntType - The uint type used for indices and more
- Vector2
- A 2-dimensional vector.
- Vector3
- A 3-dimensional vector.
- Vector4
- A 4-dimensional vector.
- Window
Size - To hold the width and height of the engine frames