Crate blue_engine
source ·Expand description
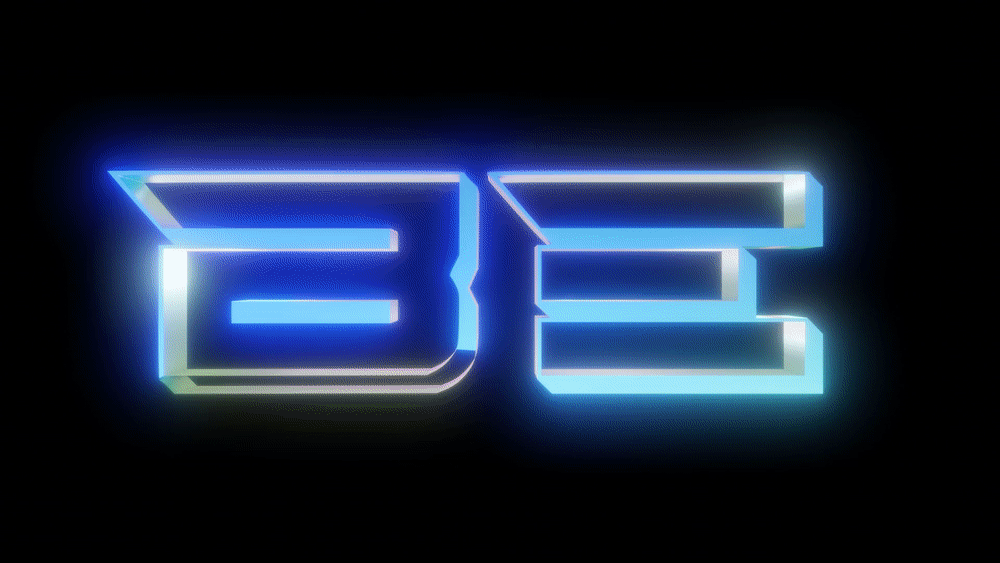
§Blue Engine
Blue Engine is an easy to use, portable, and extendable/customizable graphics engine. Here lives the documentation for the engine.
§Setup
The setup and installation details live in the project’s guide. A basic program in Blue Engine is as follow:
§Example
use blue_engine::{
header::{ Engine, ObjectSettings },
primitive_shapes::triangle
};
fn main() {
// initialize the engine
let mut engine = Engine::new().expect("engine couldn't be initialized");
// create a triangle
triangle("my triangle", ObjectSettings::default(), &mut engine.renderer, &mut engine.objects).unwrap();
// run the engine
engine
.update_loop(move |_, _, _, _, _, _| {})
.expect("Error during update loop");
}
§Utilities
This crate is the core of the engine, but there is also utilities crate which have a lot of utilities for the engine such as lighting, physics, etc.
§Guide for code navigation
The code of the engine is organized in a rather different manner than traditional in the language. There are inspirations from other languages to make it easier to navigate the project.
Re-exports§
pub use imports::*;
pub use uniform_buffer::*;
Modules§
- contains all the declarations such as structs, exports, enums, …
- re-exports from dependencies that are useful
- contains the definition for Object type, which is a type that make it easier to manage data for rendering.
- contains definition for some 2D and 3D shapes. They are basic shapes and can be used as examples of how to create your own content.
- contains definition for rendering part of the engine.
- few commonly used uniform buffer structures
- Utilities for the engine (soon moving to it’s own crate).
- contains definition for creation of window and instance creation.
Structs§
- Container for the camera feature. The settings here are needed for algebra equations needed for camera vision and movement. Please leave it to the renderer to handle
- The engine is the main starting point of using the Blue Engine. Everything that runs on Blue Engine will be under this struct. The structure of engine is monolithic, but the underlying data and the way it works is not. It gives a set of default data to work with, but also allow you to go beyond that and work as low level as you wish to.
- Instance buffer data storage
- Instance buffer data that is sent to GPU
- Objects make it easier to work with Blue Engine, it automates most of work needed for creating 3D objects and showing them on screen. A range of default objects are available as well as ability to customize each of them and even create your own! You can also customize almost everything there is about them!
- Extra settings to customize objects on time of creation
- A unified way to handle objects
- Container for pipeline values. Each pipeline takes only 1 vertex shader, 1 fragment shader, 1 texture data, and optionally a vector of uniform data.
- Main renderer class. this will contain all methods and data related to the renderer
- These definitions are taken from wgpu API docs
- Handles the live events in the engine
- Will contain all details about a vertex and will be sent to GPU
- Container for vertex and index buffer
- Descriptor and settings for a window.
Enums§
- Handles the order in which a functionality in the engine should be executed
- Container for pipeline data. Allows for sharing resources with other objects
- Container for the projection used by the camera
- Defines how the rotation axis is
- Defines how the texture data is
- Defines how the borders of texture would look like
Constants§
- Depth format
Traits§
- Allows all events to be fetched directly, making it easier to add custom additions to the engine.
- A unified way to handle strings
- A trait for StringBuffer
Functions§
- This function helps in converting pixel value to the value that is between -1 and +1