Crate shuttle_runtime
source ·Expand description
§Shuttle - Deploy Rust apps with a single Cargo subcommand
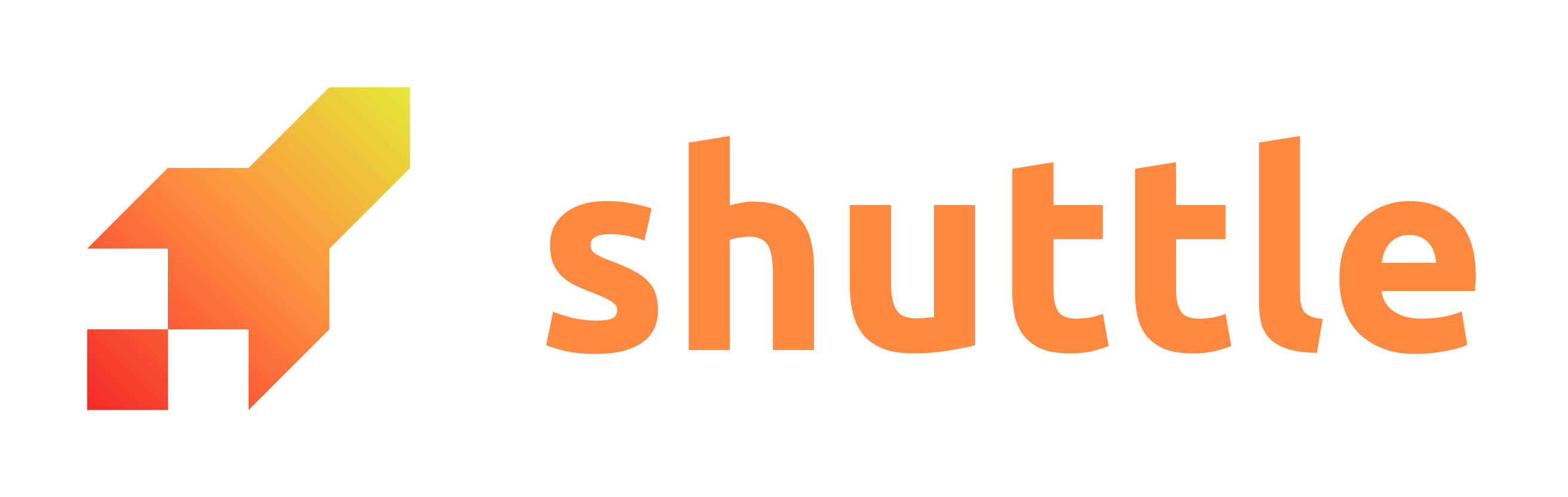
Shuttle is a Rust-native cloud development platform that lets you deploy your Rust apps for free.
📖 Check out our documentation to get started quickly: docs.shuttle.rs
🙋♂️ If you have any questions, join our Discord server.
§Usage
Start by installing the cargo shuttle
subcommand by running the following in a terminal:
cargo install cargo-shuttle
Now that Shuttle is installed, you can initialize a project with Axum boilerplate:
cargo shuttle init --template axum my-axum-app
By looking at the Cargo.toml
file of the generated my-axum-app
project you will see it has been made to
be a binary crate with a few dependencies including shuttle-runtime
and shuttle-axum
.
axum = "0.7.3"
shuttle-axum = "0.44.0"
shuttle-runtime = "0.44.0"
tokio = "1.28.2"
A boilerplate code for your axum project can also be found in src/main.rs
:
use axum::{routing::get, Router};
async fn hello_world() -> &'static str {
"Hello, world!"
}
#[shuttle_runtime::main]
async fn main() -> shuttle_axum::ShuttleAxum {
let router = Router::new().route("/", get(hello_world));
Ok(router.into())
}
Check out our docs to see all the frameworks we support, or our examples if you prefer that format.
§Running locally
To test your app locally before deploying, use:
cargo shuttle run
You should see your app build and start on the default port 8000. You can test this using;
curl http://localhost:8000/
# Hello, world!
§Deploying
Before you can deploy, you have to create a project. This will start a deployer container for your
project under the hood, ensuring isolation from other users’ projects. PS. you don’t have to do this
now if you did in in the cargo shuttle init
flow.
cargo shuttle project start
Then, deploy the service with:
cargo shuttle deploy
Your service will immediately be available at https://{project_name}.shuttleapp.rs/
. For example:
curl https://my-axum-app.shuttleapp.rs/
# Hello, world!
Re-exports§
pub use tokio;
Structs§
- The input given to Shuttle DB resources
- Shuttle Metadata
- A factory for getting metadata when building resources
- Store that holds all the secrets available to a deployment
- Shuttle Secrets
Enums§
- The environment this project is running in
- An error that can occur in the process of building and deploying a service.
Traits§
- Implement this on an
ResourceInputBuilder::Output
type to turn the base resource into the end type exposed to the Shuttle main function. - Allows implementing plugins for the Shuttle main function.
- The core trait of the Shuttle platform. Every service deployed to Shuttle needs to implement this trait.
Type Aliases§
- Type alias for an
anyhow::Error
.
Attribute Macros§
- Helper macro that generates the entrypoint required by any service - likely the only macro you need in this crate.