Crate rust_xlsxwriter_derive
source ·Expand description
The rust_xlsxwriter_derive
crate provides the XlsxSerialize
derived
trait which is used in conjunction with rust_xlsxwriter
serialization.
§Introduction
XlsxSerialize
can be used to set container and field attributes for
structs to define Excel formatting and other options when serializing them
to Excel using rust_xlsxwriter
and Serde
.
use rust_xlsxwriter::{Workbook, XlsxError, XlsxSerialize};
use serde::Serialize;
fn main() -> Result<(), XlsxError> {
let mut workbook = Workbook::new();
// Add a worksheet to the workbook.
let worksheet = workbook.add_worksheet();
// Create a serializable struct.
#[derive(XlsxSerialize, Serialize)]
#[xlsx(header_format = Format::new().set_bold())]
struct Produce {
#[xlsx(rename = "Item")]
#[xlsx(column_width = 12.0)]
fruit: &'static str,
#[xlsx(rename = "Price")]
#[xlsx(value_format = Format::new().set_num_format("$0.00"))]
cost: f64,
}
// Create some data instances.
let item1 = Produce {
fruit: "Peach",
cost: 1.05,
};
let item2 = Produce {
fruit: "Plum",
cost: 0.15,
};
let item3 = Produce {
fruit: "Pear",
cost: 0.75,
};
// Set the serialization location and headers.
worksheet.set_serialize_headers::<Produce>(0, 0)?;
// Serialize the data.
worksheet.serialize(&item1)?;
worksheet.serialize(&item2)?;
worksheet.serialize(&item3)?;
// Save the file to disk.
workbook.save("serialize.xlsx")?;
Ok(())
}
The output file is shown below. Note the change or column width in Column A, the renamed headers and the currency format in Column B numbers.
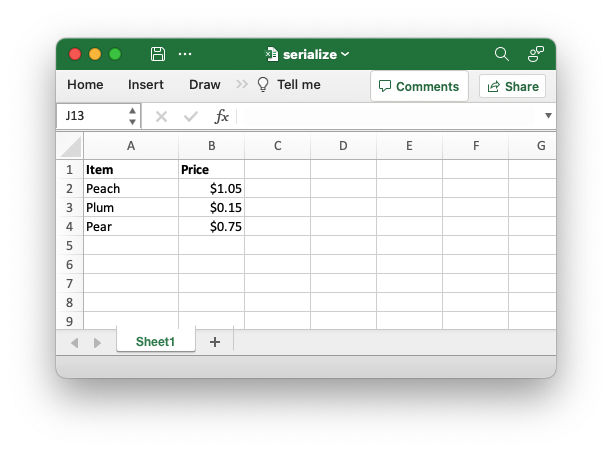
For more information see the documentation for XlsxSerialize
or Working
with Serde in the rust_xlsxwriter
docs.
Derive Macros§
- The
XlsxSerialize
derived trait is used in conjunction withrust_xlsxwriter
serialization.