Crate rune[−][src]
Expand description
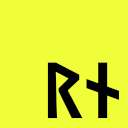
An embeddable dynamic programming language for Rust.
Contributing
If you want to help out, there should be a number of optimization tasks available in Future Optimizations. Or have a look at Open Issues.
Create an issue about the optimization you want to work on and communicate that you are working on it.
Highlights of Rune
- Clean Rust integration 💻.
- Memory safe through reference counting 📖.
- Template literals 📖.
- Try operators 📖.
- Pattern matching 📖.
- Structs and enums 📖 with associated data and functions.
- Dynamic vectors 📖, objects 📖, and tuples 📖 with built-in serde support 💻.
- First-class async support 📖.
- Generators 📖.
- Dynamic instance functions 📖.
- Stack isolation 📖 between function calls.
- Stack-based C FFI, like Lua’s (TBD).
Rune scripts
You can run Rune programs with the bundled CLI:
cargo run --bin rune -- run scripts/hello_world.rn
If you want to see detailed diagnostics of your program while it’s running, you can use:
cargo run --bin rune -- run scripts/hello_world.rn --dump-unit --trace --dump-vm
See --help
for more information.
Running scripts from Rust
You can find more examples in the
examples
folder.
The following is a complete example, including rich diagnostics using
termcolor
. It can be made much simpler if this is not needed.
use rune::{Context, Diagnostics, FromValue, Source, Sources, Vm};
use rune::termcolor::{ColorChoice, StandardStream};
use std::sync::Arc;
#[tokio::main]
async fn main() -> rune::Result<()> {
let context = Context::with_default_modules()?;
let runtime = Arc::new(context.runtime());
let mut sources = Sources::new();
sources.insert(Source::new(
"script",
r#"
pub fn add(a, b) {
a + b
}
"#,
));
let mut diagnostics = Diagnostics::new();
let result = rune::prepare(&mut sources)
.with_context(&context)
.with_diagnostics(&mut diagnostics)
.build();
if !diagnostics.is_empty() {
let mut writer = StandardStream::stderr(ColorChoice::Always);
diagnostics.emit(&mut writer, &sources)?;
}
let unit = result?;
let mut vm = Vm::new(runtime, Arc::new(unit));
let output = vm.call(&["add"], (10i64, 20i64))?;
let output = i64::from_value(output)?;
println!("{}", output);
Ok(())
}
Re-exports
pub use {{root}}::codespan_reporting::term::termcolor;
Modules
Abstract syntax trees for the Rune language.
The Rune compiler.
Diagnostics module for Rune.
The macro system of Rune.
Public packages that can be used to provide functionality to virtual machines.
Parsing utilities for Rune.
Lazy query system, used to compile and build items on demand and keep track of what’s being used and not.
Runtime module for Rune.
Macros
Helper macro to reference a specific token kind, or short sequence of kinds.
Helper macro to reference a specific token.
Helper macro to define a collection of sources populatedc with the given entries.
Structs
Error raised when we failed to load sources.
Structure to collect compilation diagnostics.
The primitive hash that among other things is used to reference items, types, and native functions.
Options that can be provided to the compiler.
A single source file.
The identifier of a source file.
A collection of source files, and a queue of things to compile.
Instructions from a single source file.
A stack which references variables indirectly from a slab.
Enums
An error raised when building the context.
An entry on the stack.
Traits
Trait used to determine what can be used as an instance function name.
Trait to handle the installation of auxilliary functions for a type installed into a module.
Helper conversion into a function hash.
Functions
Type Definitions
Boxed error type, which is an alias of anyhow::Error.
Exported result type for convenience using anyhow::Error as the default error type.