Expand description
Wavefront OBJ parser for Rust. It handles both .obj
and .mtl
formats. GitHub
use std::fs::File;
use std::io::BufReader;
use obj::{load_obj, Obj};
let input = BufReader::new(File::open("tests/fixtures/normal-cone.obj")?);
let dome: Obj = load_obj(input)?;
// Do whatever you want
dome.vertices;
dome.indices;
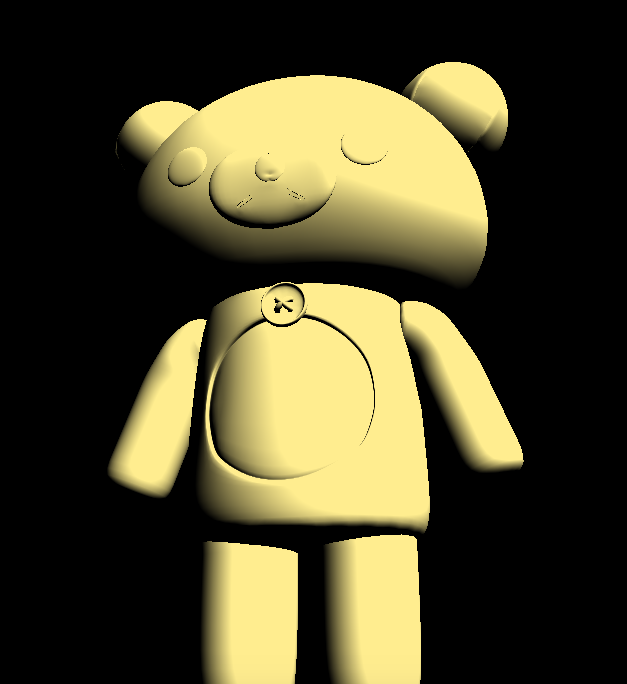
Modules§
- raw
- Provides low-level API for Wavefront OBJ format.
Structs§
- Load
Error - The error type for parse operations of the
Obj
struct. - Obj
- 3D model object loaded from wavefront OBJ.
- Position
- Vertex data type of
Obj
which contains only position data of a vertex. - Textured
Vertex - Vertex data type of
Obj
which contains position, normal and texture data of a vertex. - Vertex
- Vertex data type of
Obj
which contains position and normal data of a vertex.
Enums§
- Load
Error Kind - Enum to store the various types of errors that can cause loading an OBJ to fail.
- ObjError
- The error type for loading of the
obj
file.
Traits§
- From
RawVertex - Conversion from
RawObj
’s raw data.
Functions§
- load_
obj - Load a wavefront OBJ file into Rust & OpenGL friendly format.
Type Aliases§
- ObjResult
- A type for results generated by
load_obj
andload_mtl
where theErr
type is hard-wired toObjError