Struct rerun::SeriesPoint
source · pub struct SeriesPoint {
pub color: Option<Color>,
pub marker: Option<MarkerShape>,
pub name: Option<Name>,
pub marker_size: Option<MarkerSize>,
}
Expand description
Archetype: Define the style properties for a point series in a chart.
This archetype only provides styling information and should be logged as timeless
when possible. The underlying data needs to be logged to the same entity-path using
the Scalar
archetype.
See [Scalar
][crate::archetypes.Scalar]
§Example
§Point series
ⓘ
fn main() -> Result<(), Box<dyn std::error::Error>> {
let rec = rerun::RecordingStreamBuilder::new("rerun_example_series_point_style").spawn()?;
// Set up plot styling:
// They are logged timeless as they don't change over time and apply to all timelines.
// Log two point series under a shared root so that they show in the same plot by default.
rec.log_timeless(
"trig/sin",
&rerun::SeriesPoint::new()
.with_color([255, 0, 0])
.with_name("sin(0.01t)")
.with_marker(rerun::components::MarkerShape::Circle)
.with_marker_size(4.0),
)?;
rec.log_timeless(
"trig/cos",
&rerun::SeriesPoint::new()
.with_color([0, 255, 0])
.with_name("cos(0.01t)")
.with_marker(rerun::components::MarkerShape::Cross)
.with_marker_size(2.0),
)?;
for t in 0..((std::f32::consts::TAU * 2.0 * 10.0) as i64) {
rec.set_time_sequence("step", t);
// Log two time series under a shared root so that they show in the same plot by default.
rec.log("trig/sin", &rerun::Scalar::new((t as f64 / 10.0).sin()))?;
rec.log("trig/cos", &rerun::Scalar::new((t as f64 / 10.0).cos()))?;
}
Ok(())
}
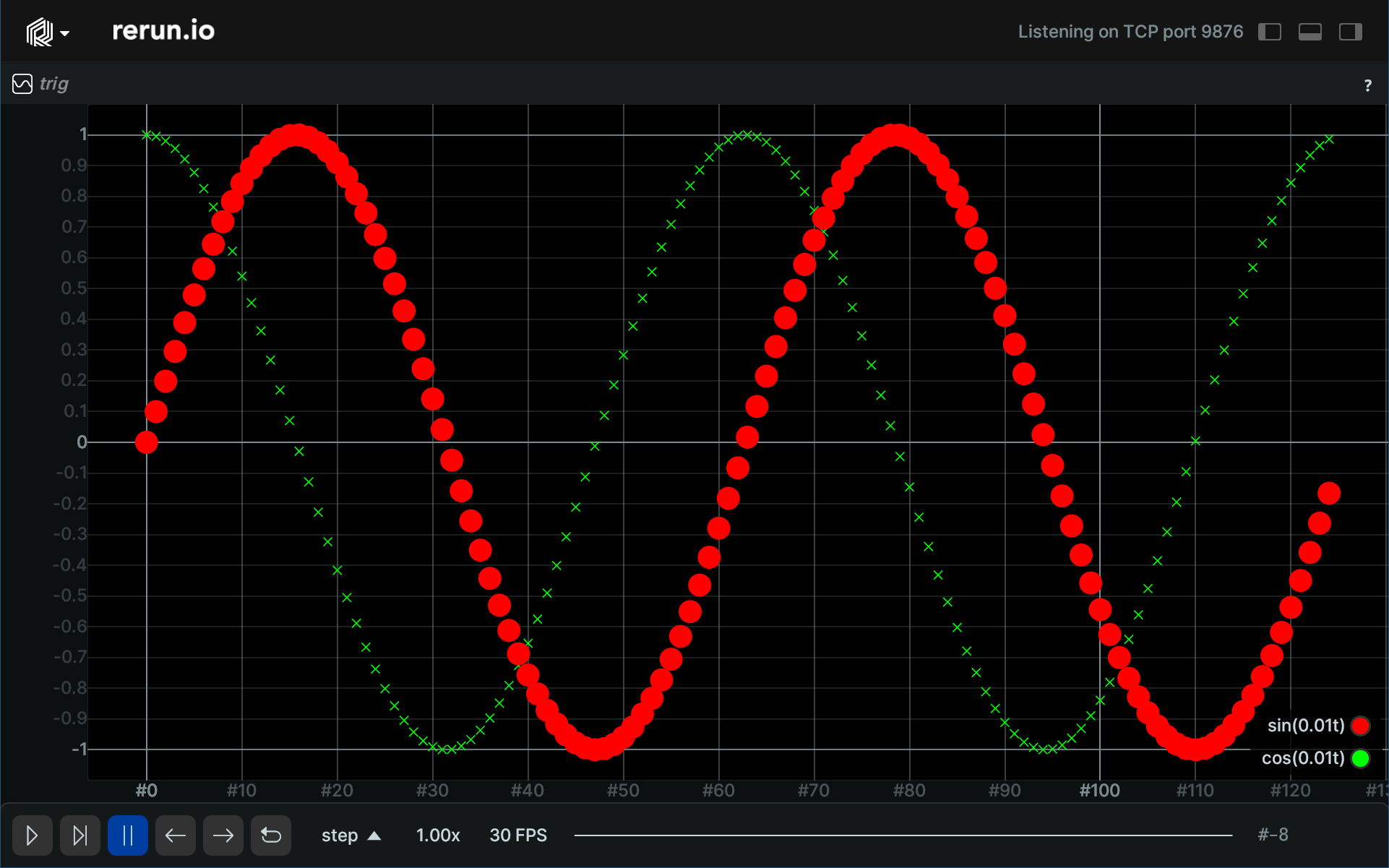
Fields§
§color: Option<Color>
Color for the corresponding series.
marker: Option<MarkerShape>
What shape to use to represent the point
name: Option<Name>
Display name of the series.
Used in the legend.
marker_size: Option<MarkerSize>
Size of the marker.
Implementations§
source§impl SeriesPoint
impl SeriesPoint
pub const NUM_COMPONENTS: usize = 6usize
source§impl SeriesPoint
impl SeriesPoint
pub fn new() -> SeriesPoint
pub fn with_color(self, color: impl Into<Color>) -> SeriesPoint
pub fn with_marker(self, marker: impl Into<MarkerShape>) -> SeriesPoint
pub fn with_name(self, name: impl Into<Name>) -> SeriesPoint
pub fn with_marker_size(self, marker_size: impl Into<MarkerSize>) -> SeriesPoint
Trait Implementations§
source§impl Archetype for SeriesPoint
impl Archetype for SeriesPoint
§type Indicator = GenericIndicatorComponent<SeriesPoint>
type Indicator = GenericIndicatorComponent<SeriesPoint>
The associated indicator component, whose presence indicates that the high-level
archetype-based APIs were used to log the data. Read more
source§fn name() -> ArchetypeName
fn name() -> ArchetypeName
The fully-qualified name of this archetype, e.g.
rerun.archetypes.Points2D
.source§fn indicator() -> MaybeOwnedComponentBatch<'static>
fn indicator() -> MaybeOwnedComponentBatch<'static>
source§fn required_components() -> Cow<'static, [ComponentName]>
fn required_components() -> Cow<'static, [ComponentName]>
Returns the names of all components that must be provided by the user when constructing
this archetype.
source§fn recommended_components() -> Cow<'static, [ComponentName]>
fn recommended_components() -> Cow<'static, [ComponentName]>
Returns the names of all components that should be provided by the user when constructing
this archetype.
source§fn optional_components() -> Cow<'static, [ComponentName]>
fn optional_components() -> Cow<'static, [ComponentName]>
Returns the names of all components that may be provided by the user when constructing
this archetype.
source§fn all_components() -> Cow<'static, [ComponentName]>
fn all_components() -> Cow<'static, [ComponentName]>
Returns the names of all components that must, should and may be provided by the user when
constructing this archetype. Read more
source§fn from_arrow_components(
arrow_data: impl IntoIterator<Item = (ComponentName, Box<dyn Array>)>
) -> Result<SeriesPoint, DeserializationError>
fn from_arrow_components( arrow_data: impl IntoIterator<Item = (ComponentName, Box<dyn Array>)> ) -> Result<SeriesPoint, DeserializationError>
Given an iterator of Arrow arrays and their respective
ComponentNames
, deserializes them
into this archetype. Read moresource§fn from_arrow(
data: impl IntoIterator<Item = (Field, Box<dyn Array>)>
) -> Result<Self, DeserializationError>where
Self: Sized,
fn from_arrow(
data: impl IntoIterator<Item = (Field, Box<dyn Array>)>
) -> Result<Self, DeserializationError>where
Self: Sized,
Given an iterator of Arrow arrays and their respective field metadata, deserializes them
into this archetype. Read more
source§impl AsComponents for SeriesPoint
impl AsComponents for SeriesPoint
source§fn as_component_batches(&self) -> Vec<MaybeOwnedComponentBatch<'_>>
fn as_component_batches(&self) -> Vec<MaybeOwnedComponentBatch<'_>>
Exposes the object’s contents as a set of
ComponentBatch
s. Read moresource§fn num_instances(&self) -> usize
fn num_instances(&self) -> usize
The number of instances in each batch. Read more
source§impl Clone for SeriesPoint
impl Clone for SeriesPoint
source§fn clone(&self) -> SeriesPoint
fn clone(&self) -> SeriesPoint
Returns a copy of the value. Read more
1.0.0 · source§fn clone_from(&mut self, source: &Self)
fn clone_from(&mut self, source: &Self)
Performs copy-assignment from
source
. Read moresource§impl Debug for SeriesPoint
impl Debug for SeriesPoint
source§impl SizeBytes for SeriesPoint
impl SizeBytes for SeriesPoint
source§fn heap_size_bytes(&self) -> u64
fn heap_size_bytes(&self) -> u64
Returns the total size of
self
on the heap, in bytes.source§fn total_size_bytes(&self) -> u64
fn total_size_bytes(&self) -> u64
Returns the total size of
self
in bytes, accounting for both stack and heap space.source§fn stack_size_bytes(&self) -> u64
fn stack_size_bytes(&self) -> u64
Returns the total size of
self
on the stack, in bytes. Read moreAuto Trait Implementations§
impl Freeze for SeriesPoint
impl RefUnwindSafe for SeriesPoint
impl Send for SeriesPoint
impl Sync for SeriesPoint
impl Unpin for SeriesPoint
impl UnwindSafe for SeriesPoint
Blanket Implementations§
source§impl<T> BorrowMut<T> for Twhere
T: ?Sized,
impl<T> BorrowMut<T> for Twhere
T: ?Sized,
source§fn borrow_mut(&mut self) -> &mut T
fn borrow_mut(&mut self) -> &mut T
Mutably borrows from an owned value. Read more
source§impl<T> CheckedAs for T
impl<T> CheckedAs for T
source§fn checked_as<Dst>(self) -> Option<Dst>where
T: CheckedCast<Dst>,
fn checked_as<Dst>(self) -> Option<Dst>where
T: CheckedCast<Dst>,
Casts the value.
source§impl<Src, Dst> CheckedCastFrom<Src> for Dstwhere
Src: CheckedCast<Dst>,
impl<Src, Dst> CheckedCastFrom<Src> for Dstwhere
Src: CheckedCast<Dst>,
source§fn checked_cast_from(src: Src) -> Option<Dst>
fn checked_cast_from(src: Src) -> Option<Dst>
Casts the value.
source§impl<T> Downcast for Twhere
T: Any,
impl<T> Downcast for Twhere
T: Any,
source§fn into_any(self: Box<T>) -> Box<dyn Any>
fn into_any(self: Box<T>) -> Box<dyn Any>
Convert
Box<dyn Trait>
(where Trait: Downcast
) to Box<dyn Any>
. Box<dyn Any>
can
then be further downcast
into Box<ConcreteType>
where ConcreteType
implements Trait
.source§fn into_any_rc(self: Rc<T>) -> Rc<dyn Any>
fn into_any_rc(self: Rc<T>) -> Rc<dyn Any>
Convert
Rc<Trait>
(where Trait: Downcast
) to Rc<Any>
. Rc<Any>
can then be
further downcast
into Rc<ConcreteType>
where ConcreteType
implements Trait
.source§fn as_any(&self) -> &(dyn Any + 'static)
fn as_any(&self) -> &(dyn Any + 'static)
Convert
&Trait
(where Trait: Downcast
) to &Any
. This is needed since Rust cannot
generate &Any
’s vtable from &Trait
’s.source§fn as_any_mut(&mut self) -> &mut (dyn Any + 'static)
fn as_any_mut(&mut self) -> &mut (dyn Any + 'static)
Convert
&mut Trait
(where Trait: Downcast
) to &Any
. This is needed since Rust cannot
generate &mut Any
’s vtable from &mut Trait
’s.source§impl<T> DowncastSync for T
impl<T> DowncastSync for T
source§impl<T> Instrument for T
impl<T> Instrument for T
source§fn instrument(self, span: Span) -> Instrumented<Self>
fn instrument(self, span: Span) -> Instrumented<Self>
source§fn in_current_span(self) -> Instrumented<Self>
fn in_current_span(self) -> Instrumented<Self>
source§impl<Src, Dst> LosslessTryInto<Dst> for Srcwhere
Dst: LosslessTryFrom<Src>,
impl<Src, Dst> LosslessTryInto<Dst> for Srcwhere
Dst: LosslessTryFrom<Src>,
source§fn lossless_try_into(self) -> Option<Dst>
fn lossless_try_into(self) -> Option<Dst>
Performs the conversion.
source§impl<Src, Dst> LossyInto<Dst> for Srcwhere
Dst: LossyFrom<Src>,
impl<Src, Dst> LossyInto<Dst> for Srcwhere
Dst: LossyFrom<Src>,
source§fn lossy_into(self) -> Dst
fn lossy_into(self) -> Dst
Performs the conversion.
source§impl<T> OverflowingAs for T
impl<T> OverflowingAs for T
source§fn overflowing_as<Dst>(self) -> (Dst, bool)where
T: OverflowingCast<Dst>,
fn overflowing_as<Dst>(self) -> (Dst, bool)where
T: OverflowingCast<Dst>,
Casts the value.
source§impl<Src, Dst> OverflowingCastFrom<Src> for Dstwhere
Src: OverflowingCast<Dst>,
impl<Src, Dst> OverflowingCastFrom<Src> for Dstwhere
Src: OverflowingCast<Dst>,
source§fn overflowing_cast_from(src: Src) -> (Dst, bool)
fn overflowing_cast_from(src: Src) -> (Dst, bool)
Casts the value.
source§impl<T> Pointable for T
impl<T> Pointable for T
source§impl<T> SaturatingAs for T
impl<T> SaturatingAs for T
source§fn saturating_as<Dst>(self) -> Dstwhere
T: SaturatingCast<Dst>,
fn saturating_as<Dst>(self) -> Dstwhere
T: SaturatingCast<Dst>,
Casts the value.
source§impl<Src, Dst> SaturatingCastFrom<Src> for Dstwhere
Src: SaturatingCast<Dst>,
impl<Src, Dst> SaturatingCastFrom<Src> for Dstwhere
Src: SaturatingCast<Dst>,
source§fn saturating_cast_from(src: Src) -> Dst
fn saturating_cast_from(src: Src) -> Dst
Casts the value.
source§impl<T> UnwrappedAs for T
impl<T> UnwrappedAs for T
source§fn unwrapped_as<Dst>(self) -> Dstwhere
T: UnwrappedCast<Dst>,
fn unwrapped_as<Dst>(self) -> Dstwhere
T: UnwrappedCast<Dst>,
Casts the value.
source§impl<Src, Dst> UnwrappedCastFrom<Src> for Dstwhere
Src: UnwrappedCast<Dst>,
impl<Src, Dst> UnwrappedCastFrom<Src> for Dstwhere
Src: UnwrappedCast<Dst>,
source§fn unwrapped_cast_from(src: Src) -> Dst
fn unwrapped_cast_from(src: Src) -> Dst
Casts the value.
source§impl<T> WithSubscriber for T
impl<T> WithSubscriber for T
source§fn with_subscriber<S>(self, subscriber: S) -> WithDispatch<Self>
fn with_subscriber<S>(self, subscriber: S) -> WithDispatch<Self>
source§fn with_current_subscriber(self) -> WithDispatch<Self>
fn with_current_subscriber(self) -> WithDispatch<Self>
source§impl<T> WrappingAs for T
impl<T> WrappingAs for T
source§fn wrapping_as<Dst>(self) -> Dstwhere
T: WrappingCast<Dst>,
fn wrapping_as<Dst>(self) -> Dstwhere
T: WrappingCast<Dst>,
Casts the value.
source§impl<Src, Dst> WrappingCastFrom<Src> for Dstwhere
Src: WrappingCast<Dst>,
impl<Src, Dst> WrappingCastFrom<Src> for Dstwhere
Src: WrappingCast<Dst>,
source§fn wrapping_cast_from(src: Src) -> Dst
fn wrapping_cast_from(src: Src) -> Dst
Casts the value.