Expand description
§SeaORM
§SeaORM is a relational ORM to help you build web services in Rust with the familiarity of dynamic languages.
If you like what we do, consider starring, sharing and contributing!
Please help us with maintaining SeaORM by completing the SeaQL Community Survey 2024!
§Getting Started
Integration examples:
- Actix v4 Example
- Actix v3 Example
- Axum Example
- GraphQL Example
- jsonrpsee Example
- Poem Example
- Rocket Example
- Salvo Example
- Tonic Example
- Seaography Example
§Support
Join our Discord server to chat with other members of the SeaQL community!
Professional support on Rust programming and best practices is available. You can email us for a quote!
§Features
-
Async
Relying on SQLx, SeaORM is a new library with async support from day 1.
-
Dynamic
Built upon SeaQuery, SeaORM allows you to build complex dynamic queries.
-
Testable
Use mock connections and/or SQLite to write tests for your application logic.
-
Service Oriented
Quickly build services that join, filter, sort and paginate data in REST, GraphQL and gRPC APIs.
§A quick taste of SeaORM
§Entity
use sea_orm::entity::prelude::*;
#[derive(Clone, Debug, PartialEq, DeriveEntityModel)]
#[sea_orm(table_name = "cake")]
pub struct Model {
#[sea_orm(primary_key)]
pub id: i32,
pub name: String,
}
#[derive(Copy, Clone, Debug, EnumIter, DeriveRelation)]
pub enum Relation {
#[sea_orm(has_many = "super::fruit::Entity")]
Fruit,
}
impl Related<super::fruit::Entity> for Entity {
fn to() -> RelationDef {
Relation::Fruit.def()
}
}
§Select
// find all models
let cakes: Vec<cake::Model> = Cake::find().all(db).await?;
// find and filter
let chocolate: Vec<cake::Model> = Cake::find()
.filter(cake::Column::Name.contains("chocolate"))
.all(db)
.await?;
// find one model
let cheese: Option<cake::Model> = Cake::find_by_id(1).one(db).await?;
let cheese: cake::Model = cheese.unwrap();
// find related models (lazy)
let fruits: Vec<fruit::Model> = cheese.find_related(Fruit).all(db).await?;
// find related models (eager)
let cake_with_fruits: Vec<(cake::Model, Vec<fruit::Model>)> =
Cake::find().find_with_related(Fruit).all(db).await?;
§Insert
let apple = fruit::ActiveModel {
name: Set("Apple".to_owned()),
..Default::default() // no need to set primary key
};
let pear = fruit::ActiveModel {
name: Set("Pear".to_owned()),
..Default::default()
};
// insert one
let pear = pear.insert(db).await?;
// insert many
Fruit::insert_many([apple, pear]).exec(db).await?;
§Update
use sea_orm::sea_query::{Expr, Value};
let pear: Option<fruit::Model> = Fruit::find_by_id(1).one(db).await?;
let mut pear: fruit::ActiveModel = pear.unwrap().into();
pear.name = Set("Sweet pear".to_owned());
// update one
let pear: fruit::Model = pear.update(db).await?;
// update many: UPDATE "fruit" SET "cake_id" = NULL WHERE "fruit"."name" LIKE '%Apple%'
Fruit::update_many()
.col_expr(fruit::Column::CakeId, Expr::value(Value::Int(None)))
.filter(fruit::Column::Name.contains("Apple"))
.exec(db)
.await?;
§Save
let banana = fruit::ActiveModel {
id: NotSet,
name: Set("Banana".to_owned()),
..Default::default()
};
// create, because primary key `id` is `NotSet`
let mut banana = banana.save(db).await?;
banana.name = Set("Banana Mongo".to_owned());
// update, because primary key `id` is `Set`
let banana = banana.save(db).await?;
§Delete
// delete one
let orange: Option<fruit::Model> = Fruit::find_by_id(1).one(db).await?;
let orange: fruit::Model = orange.unwrap();
fruit::Entity::delete(orange.into_active_model())
.exec(db)
.await?;
// or simply
let orange: Option<fruit::Model> = Fruit::find_by_id(1).one(db).await?;
let orange: fruit::Model = orange.unwrap();
orange.delete(db).await?;
// delete many: DELETE FROM "fruit" WHERE "fruit"."name" LIKE 'Orange'
fruit::Entity::delete_many()
.filter(fruit::Column::Name.contains("Orange"))
.exec(db)
.await?;
§đ§ Seaography: GraphQL integration (preview)
Seaography is a GraphQL framework built on top of SeaORM. Seaography allows you to build GraphQL resolvers quickly. With just a few commands, you can launch a GraphQL server from SeaORM entities!
Starting 0.12
, seaography
integration is built into sea-orm
. While Seaography development is still in an early stage, it is especially useful in prototyping and building internal-use admin panels.
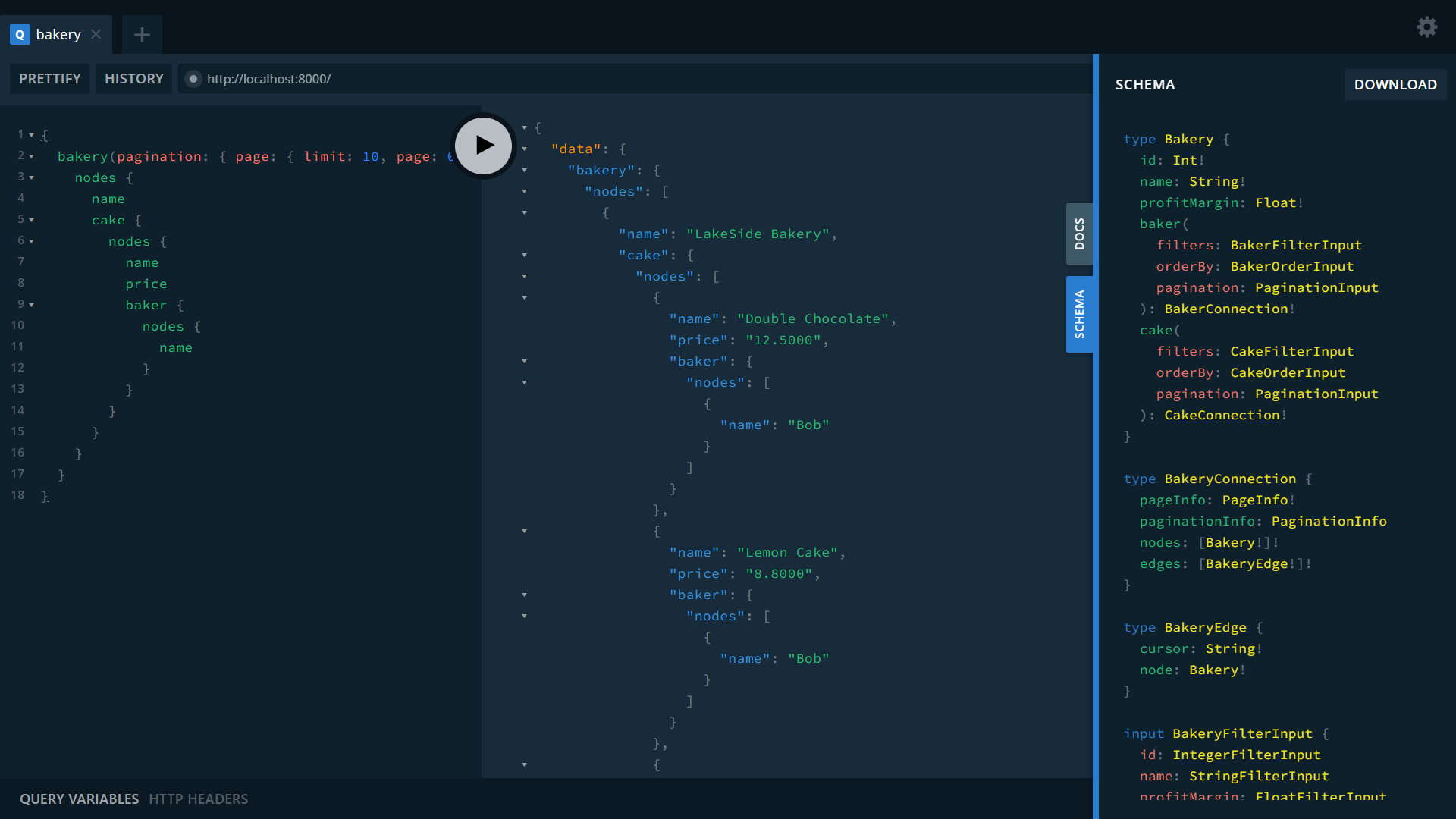
Look at the Seaography Example to learn more.
§Learn More
§Whoâs using SeaORM?
See Built with SeaORM. Feel free to submit yours!
§License
Licensed under either of
- Apache License, Version 2.0 (LICENSE-APACHE or http://www.apache.org/licenses/LICENSE-2.0)
- MIT license (LICENSE-MIT or http://opensource.org/licenses/MIT)
at your option.
§Contribution
Unless you explicitly state otherwise, any contribution intentionally submitted for inclusion in the work by you, as defined in the Apache-2.0 license, shall be dual licensed as above, without any additional terms or conditions.
SeaORM is a community driven project. We welcome you to participate, contribute and together help build Rustâs future.
A big shout out to our contributors!
§Sponsorship
SeaQL.org is an independent open-source organization run by passionate developers. If you enjoy using our libraries, please star and share our repositories. If you feel generous, a small donation via GitHub Sponsor will be greatly appreciated, and goes a long way towards sustaining the organization.
We invite you to participate, contribute and together help build Rustâs future.
§Gold Sponsors
§Mascot
A friend of Ferris, Terres the hermit crab is the official mascot of SeaORM. His hobby is collecting shells.
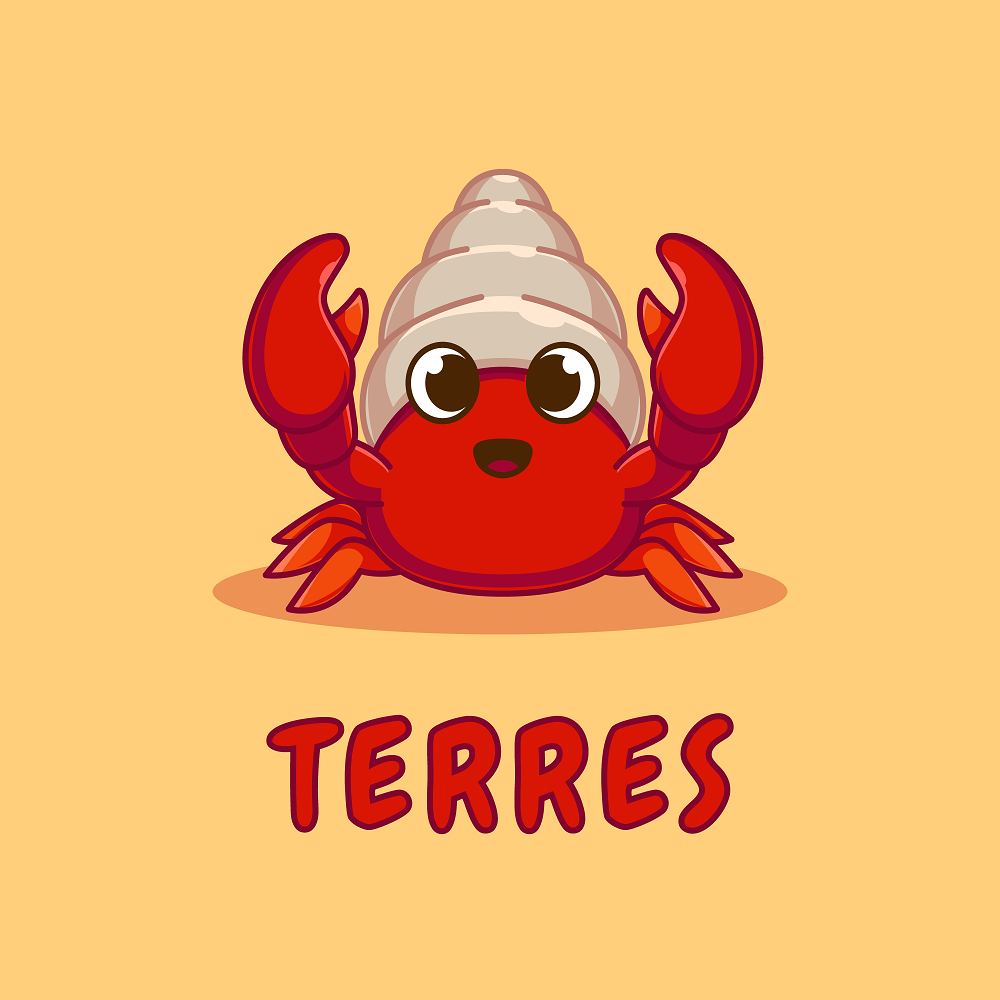
Re-exports§
pub use sea_query;
pub use strum;
pub use entity::*;
pub use error::*;
pub use query::*;
pub use schema::*;
Modules§
- Module for the Entity type and operations
- Error types for all database operations
- Holds types and methods to perform metric collection
- Holds types and methods to perform queries
- Holds types that defines the schemas of an Entity
Macros§
- Non-debug version
- Helper to get a raw SQL string from an object that impl
QueryTrait
. - Helper to get a
Statement
from an object that implQueryTrait
.
Structs§
- Defines the configuration options of a database
- Cursor pagination
- Defines a database
- Defines a database transaction, whether it is an open transaction and the type of backend to use
- The result of a DELETE operation
- Handles DELETE operations in a ActiveModel using DeleteStatement
- Defines the result of executing an operation
- The result of an INSERT operation on an ActiveModel
- Defines a structure to perform INSERT operations in an ActiveModel
- Define a structure containing the numbers of items and pages of a Paginator
- MockDatabase
mock
Defines a Mock database suitable for testing - Defines a connection for the MockDatabase
- Defines a database driver for the MockDatabase
- MockExecResult
mock
Defines the results obtained from a MockDatabase - MockRow
mock
- OpenTransaction
mock
Defines a transaction that is has not been committed - Defined a structure to handle pagination of a result from a query operation on a Model
- Defines a connection for the [ProxyDatabase]
- Defines a database driver for the [ProxyDatabase]
- ProxyExecResult
proxy
Defines the results obtained from a [ProxyDatabase] - ProxyRow
proxy
- Defines the result of a query operation on a Model
- The self-referencing struct.
- Get tuple from query result based on column index
- Get tuple from query result based on a list of column identifiers
- Defines a type to get a Model
- Defines a type to get two Models
- Defines a type to do
SELECT
operations through a SelectStatement on a Model - Performs a raw
SELECT
operation on a model - Defines the sqlx::mysql connector
- Defines a sqlx MySQL pool
- Defines the sqlx::postgres connector
- Defines a sqlx PostgreSQL pool
- Defines the sqlx::sqlite connector
- Defines a sqlx SQLite pool
- Defines an SQL statement
- Transaction
mock
Defines a database transaction as it holds a Vec<Statement> - The self-referencing struct.
- The result of an update operation on an ActiveModel
- Defines an update operation
Enums§
- Access mode
- The type of database backend for real world databases. This is enabled by feature flags as specified in the crate documentation
- Handle a database connection depending on the backend enabled by the feature flags. This creates a database pool.
- Isolation level
- Defines errors for handling transaction failures
- An error from trying to get a row from a Model
- The types of results for an INSERT operation
- Value variants
Traits§
- The generic API for a database connection that can perform query or execute statements. It abstracts database connection and transaction
- A trait for any type that can be turn into a cursor
- Identifier
- IntoMockRow
mock
A trait to get a MockRow from a type useful for testing in the MockDatabase - A Trait for any type wanting to perform operations on the MockDatabase
- A Trait for any type that can paginate results
- ProxyDatabaseTrait
proxy
Defines the ProxyDatabaseTrait to save the functions - A Trait for any type that can perform SELECT queries
- Any type that can build a Statement
- Stream query results
- Spawn database transaction
- Try to convert a type to a u64
- An interface to get a value from the query result
- An interface to get an array of values from the query result. A type can only implement
ActiveEnum
orTryGetableFromJson
, but not both. A blanket impl is provided forTryGetableFromJson
, while the impl forActiveEnum
is provided by theDeriveActiveEnum
macro. So as an end user you wonât normally touch this trait. - An interface to get a JSON from the query result
- An interface to get a tuple value from the query result
Functions§
- Convert QueryResult to ProxyRow
- Converts an sqlx::error connection error to a DbErr
- Converts an sqlx::error execution error to a DbErr
- Converts an sqlx::error query error to a DbErr
Type Aliases§
- The same as DatabaseBackend just shorter :)
- The same as a DatabaseConnection
- Pin a Model so that stream operations can be performed on the model
Derive Macros§
- A derive macro to implement
sea_orm::ActiveEnum
trait for enums. - The DeriveActiveModel derive macro will implement ActiveModelTrait for ActiveModel which provides setters and getters for all active values in the active model.
- Models that a user can override
- The DeriveColumn derive macro will implement [ColumnTrait] for Columns. It defines the identifier of each column by implementing Iden and IdenStatic. The EnumIter is also derived, allowing iteration over all enum variants.
- Derive a column if column names are not in snake-case
- Create an Entity
- This derive macro is the âalmightyâ macro which automatically generates Entity, Column, and PrimaryKey from a given Model.
- The DeriveIden derive macro will implement
sea_orm::sea_query::Iden
for simplify Iden implementation. - Derive into an active model
- The DeriveMigrationName derive macro will implement
sea_orm_migration::MigrationName
for a migration. - The DeriveModel derive macro will implement ModelTrait for Model, which provides setters and getters for all attributes in the mod It also implements FromQueryResult to convert a query result into the corresponding Model.
- The DerivePartialModel derive macro will implement
sea_orm::PartialModelTrait
for simplify partial model queries. - The DerivePrimaryKey derive macro will implement [PrimaryKeyToColumn] for PrimaryKey which defines tedious mappings between primary keys and columns. The EnumIter is also derived, allowing iteration over all enum variants.
- The DeriveRelatedEntity derive macro will implement seaography::RelationBuilder for RelatedEntity enumeration.
- The DeriveRelation derive macro will implement RelationTrait for Relation.
- Implements traits for types that wrap a database value type.
- Creates a new type that iterates of the variants of an enum.
- Convert a query result into the corresponding Model.