1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102
//! # Crate ray_tracing_core //! //! GitHub page [rabbid76.github.io/ray-tracing-with-rust](https://rabbid76.github.io/ray-tracing-with-rust/) //! GitHub repository [Rabbid76/ray-tracing-with-rust](https://github.com/Rabbid76/ray-tracing-with-rust) //! //! [](https://stackoverflow.com/users/5577765/rabbid76?tab=profile) //! //! Based on [Peter Shirley's](https://research.nvidia.com/person/peter-shirley) books: //! //! - ["Ray Tracing in One Weekend (Ray Tracing Minibooks Book 1)"](https://raytracing.github.io/books/RayTracingInOneWeekend.html) //! - ["Ray Tracing: the Next Week (Ray Tracing Minibooks Book 2)"](https://raytracing.github.io/books/RayTracingTheNextWeek.html) //! - ["Ray Tracing: The Rest of Your Life (Ray Tracing Minibooks Book 3)"](https://raytracing.github.io/books/RayTracingTheRestOfYourLife.html) //! //! 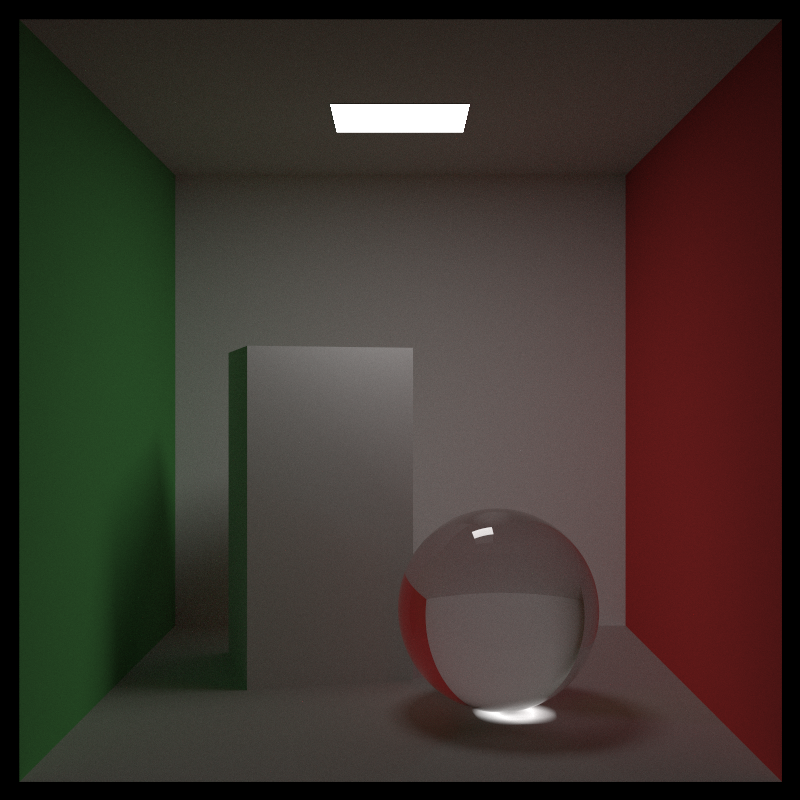 //! //! “Note that I avoid most “modern features” of C++, but inheritance and operator overloading are too useful for ray tracers to pass on.” //! ― [Peter Shirley](https://research.nvidia.com/person/peter-shirley), [Ray Tracing in One Weekend](https://www.goodreads.com/book/show/28794030-ray-tracing-in-one-weekend) //! //! # Example //! //! ```rust //! use ray_tracing_core::random; //! use ray_tracing_core::test::TestSceneSimple; //! use ray_tracing_core::types::ColorRGB; //! use ray_tracing_core::types::FSize; //! //! fn main() { //! let cx = 40; //! let cy = 20; //! let samples = 10; //! let scene = TestSceneSimple::new().scene; //! //! let mut pixel_data: Vec<u8> = Vec::with_capacity(cx * cy * 4); //! pixel_data.resize(cx * cy * 4, 0); //! //! for y in 0..cy { //! for x in 0..cx { //! let mut c = ColorRGB::new(0.0, 0.0, 0.0); //! for _ in 0..samples { //! let u = (x as FSize + random::generate_size()) / cx as FSize; //! let v = 1.0 - (y as FSize + random::generate_size()) / cy as FSize; //! c = c + scene.ray_trace_color(u, v); //! } //! c = c / samples as FSize; //! //! let i = (y * cx) + x; //! pixel_data[i * 4] = (c[0].sqrt() * 255.0).round() as u8; //! pixel_data[i * 4 + 1] = (c[1].sqrt() * 255.0).round() as u8; //! pixel_data[i * 4 + 2] = (c[2].sqrt() * 255.0).round() as u8; //! pixel_data[i * 4 + 3] = 255; //! } //! } //! //! // [...] //! } //! ``` /// Ray tracing data types /// /// Implementation of the data types used for the ray tracing calculations using [Crate `glm`](https://docs.rs/glm/0.2.3/glm/index.html) pub mod types; /// Random data generator /// /// Generators for random data like vectors and colors using [Crate `rand`](https://docs.rs/rand/0.8.3/rand/) pub mod random; /// Ray Trace Math /// /// Ray trace math objects and equations pub mod math; /// Texture objects /// /// Implementation of ray tracing textures pub mod texture; /// Material objects /// /// Implementation of ray tracing materials pub mod material; /// Hit able objects /// /// Implementation of ray tracing hit ables pub mod hit_able; /// Environment /// /// Implementation of environment like sky pub mod environment; /// Ray Trace core /// /// Ray trace core objects pub mod core; /// Probability Density Function pub mod probability_density_function; /// Internal module for test for integration tests pub mod test;