1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79
//! The definitive vector and matrix library for Rust //! //! # In short //! 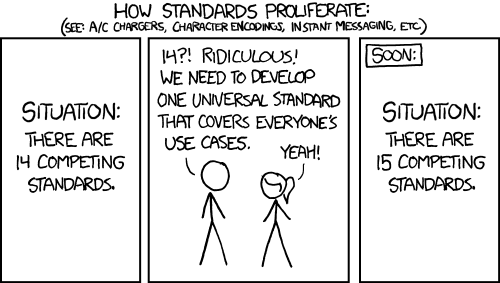 //! //! My take on a vector library, that tries to be the be-all end-all vector and //! matrix crate through hand written SIMD for games and const generics for //! scientific compute. //! //! In order to achieve this, `definitive` relies on experimental Rust features, //! such as const generics and specialization. This puts `definitive` in the //! experimental category, as it may cause internal compiler errors or undefined //! behaviour. //! //! # Matrices? //! You might ponder, for a vector and **matrix** library, `definitive` doesn't //! seem to have any matrices! This is an unfortunate issue that I am currently //! facing with const generics that should be resolved in the future. Before //! support in rustc matures, `definitive` will support "good enough" matrices //! that aren't const sized. //! //! # Example //! ```rust //! use definitive::Vector; //! //! let a = Vector::from([2, 3, 1]); //! let b = Vector::from([8, 0, 0]); //! //! dbg!(a + b * 4); //! // [34, 3, 1] //! ``` //! //! ### `#![no_std]` is supported by disabling the `std` feature #![feature(const_generics, specialization, trivial_bounds)] #![allow(incomplete_features)] #![deny(missing_docs)] #![cfg_attr(not(feature = "std"), no_std)] mod add; mod add_assign; mod clone; mod copy; mod debug; mod deref; mod div; mod div_assign; mod eq; mod macros; mod mul; mod mul_assign; mod neg; mod partial_eq; mod rem; mod rem_assign; mod sub; mod sub_assign; mod traits; mod vector; pub use traits::{One, Sqrt, Zero}; /// Shorthand for Vector<f32, 2> pub type Vec2 = Vector<f32, 2>; /// Shorthand for Vector<f32, 3> pub type Vec3 = Vector<f32, 3>; /// Shorthand for Vector<f32, 4> pub type Vec4 = Vector<f32, 4>; /// A generic type representing an N dimensional vector pub struct Vector<T = f32, const N: usize>([T; { N }]); impl<T, const N: usize> From<[T; { N }]> for Vector<T, { N }> { fn from(v: [T; { N }]) -> Self { Self(v) } } // TODO: Figure out a workaround // struct Matrix<T, const N: usize, const D: [usize; N]>([T; { D.iter().sum() }]);