1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97
// lib.rs Pix crate. // // Copyright (c) 2019-2020 Douglas P Lau // Copyright (c) 2019-2020 Jeron Aldaron Lau // //! Library for pixel and image compositing. //! //! A [raster] image is a rectangular array of [pixel]s. //! //! ## Color Models //! * `RGB` / `BGR` (*red*, *green*, *blue*) //! * `Gray` (*luma* / *relative luminance*) //! * `HSV` (*hue*, *saturation*, *value*) //! * `HSL` (*hue*, *saturation*, *lightness*) //! * `HWB` (*hue*, *whiteness*, *blackness*) //! * `YCbCr` (JPEG) //! * `Matte` (*alpha* only) //! //! [alpha]: chan/trait.Alpha.html //! [channel]: chan/trait.Channel.html //! [color model]: clr/trait.ColorModel.html //! [gamma]: chan/trait.Gamma.html //! [pixel]: el/trait.Pixel.html //! [raster]: struct.Raster.html //! //! ### Example: Color Demo //! ``` //! use pix::{Raster, SHwb8}; //! //! let mut r = Raster::<SHwb8>::with_clear(256, 256); //! for (y, row) in r.rows_mut().enumerate() { //! for (x, p) in row.iter_mut().enumerate() { //! let h = ((x + y) >> 1) as u8; //! let w = y.saturating_sub(x) as u8; //! let b = x.saturating_sub(y) as u8; //! *p = SHwb8::new(h, w, b); //! } //! } //! ``` //! //! 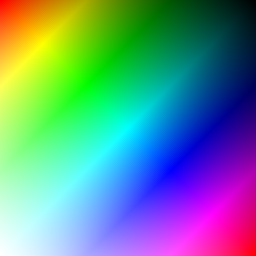 //! //! ### Example: Convert Raster Format //! ``` //! use pix::{Raster, Rgba8p, SRgb8}; //! //! let mut src = Raster::<SRgb8>::with_clear(120, 120); //! // ... load pixels into raster //! let dst: Raster<Rgba8p> = Raster::with_raster(&src); //! ``` //! //! ## Documentation //! [https://docs.rs/pix](https://docs.rs/pix) //! #![warn(missing_docs)] #![warn(missing_doc_code_examples)] pub mod chan; pub mod clr; pub mod el; pub mod ops; mod palette; mod private; mod raster; pub use crate::clr::bgr::{ Bgr16, Bgr32, Bgr8, Bgra16, Bgra16p, Bgra32, Bgra32p, Bgra8, Bgra8p, SBgr16, SBgr32, SBgr8, SBgra16, SBgra16p, SBgra32, SBgra32p, SBgra8, SBgra8p, }; pub use crate::clr::gray::{ Gray16, Gray32, Gray8, Graya16, Graya16p, Graya32, Graya32p, Graya8, Graya8p, SGray16, SGray32, SGray8, SGraya16, SGraya16p, SGraya32, SGraya32p, SGraya8, SGraya8p, }; pub use crate::clr::hsl::{ Hsl16, Hsl32, Hsl8, Hsla16, Hsla16p, Hsla32, Hsla32p, Hsla8, Hsla8p, }; pub use crate::clr::hsv::{ Hsv16, Hsv32, Hsv8, Hsva16, Hsva16p, Hsva32, Hsva32p, Hsva8, Hsva8p, }; pub use crate::clr::hwb::{ Hwb16, Hwb32, Hwb8, Hwba16, Hwba16p, Hwba32, Hwba32p, Hwba8, Hwba8p, SHwb16, SHwb32, SHwb8, }; pub use crate::clr::matte::{Matte16, Matte32, Matte8}; pub use crate::clr::rgb::{ Rgb16, Rgb32, Rgb8, Rgba16, Rgba16p, Rgba32, Rgba32p, Rgba8, Rgba8p, SRgb16, SRgb32, SRgb8, SRgba16, SRgba16p, SRgba32, SRgba32p, SRgba8, SRgba8p, }; pub use crate::clr::ycc::{ YCbCr16, YCbCr32, YCbCr8, YCbCra16, YCbCra16p, YCbCra32, YCbCra32p, YCbCra8, YCbCra8p, }; pub use crate::palette::Palette; pub use crate::raster::{Raster, Region, Rows, RowsMut};